Introduction
Sentiment analysis, also known as opinion mining, is a powerful concept in the Natural Language Processing (NLP) technique that interprets and classifies emotions expressed in textual data. Of course, it identifies whether the sentiment is positive, negative, or neutral. With the outcome, each business and researcher can enable and understand customer opinions, market trends, and social attitudes. By analyzing data collected from sources like social media, reviews, and surveys, sentiment analysis provides powerful, actionable insights, driving decision-making, enhancing customer satisfaction, and optimizing strategies across industries to enable the business. This technology helps bridge the gap between raw data and meaningful emotional context, making it a cornerstone in the era of data-driven innovation.
In this article, we will explore how sentiment analysis at scale is essential for analyzing vast amounts of text data in diverse languages and specialized domains, how it enables businesses and researchers to uncover real-time insights into customer sentiment, market trends, and user experiences, spanning global markets and industry-specific contexts. This capability is achieved by combining advanced Natural Language Processing (NLP) techniques, scalable technologies, and deep learning models.
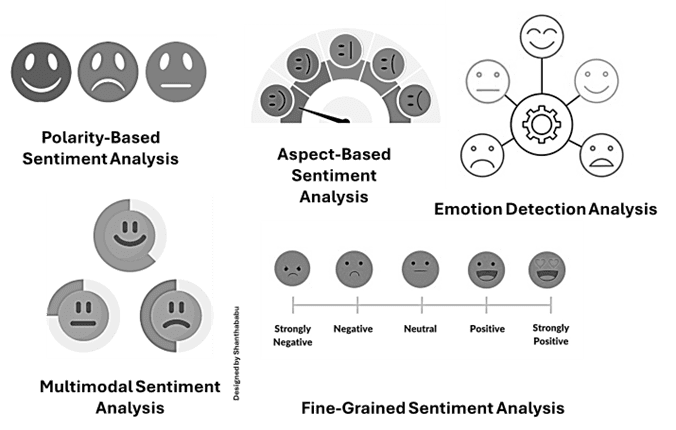
Key concepts in sentiment analysis
Let’s understand the key concepts in sentiment analysis,
Multi-lingual sentiment analysis:
This analysis is highly demanded due to the global market and business enhancement. Multi-lingual sentiment analysis extends the capabilities of traditional sentiment analysis by interpreting and categorizing emotions across multiple languages in the global market. Leveraging advanced natural language processing (NLP) models and translation tools enable businesses and researchers to understand global customer sentiments, market dynamics, and cultural nuances. This approach is essential for organizations operating in diverse regions, as it provides inclusive insights, enhances customer engagement, and ensures accurate sentiment interpretation without language barriers. Multi-lingual sentiment analysis empowers global strategies, bridging linguistic diversity with actionable emotional intelligence.
- Use cases of multi-lingual sentiment analysis:
- Global Brand Monitoring: Analyze customer feedback, reviews, and social media sentiment across different languages to track brand reputation worldwide.
- Example: Identifying regional customer satisfaction trends for an international product launch.
- E-commerce and Retail Insights: Assess customer reviews in multiple languages to improve product offerings, supply chain management, and personalized recommendations.Example: Analyzing product reviews in diverse markets to optimize stock and improve product descriptions.
- Global Brand Monitoring: Analyze customer feedback, reviews, and social media sentiment across different languages to track brand reputation worldwide.
- Techniques:
- Pre-Trained Multi-Lingual Models: Multilingual Language Models are a subset of Natural Language Processing models pre-trained on text data from various languages to accommodate the needs of the demand. Many models available, like XLM-Roberta and mBERT, provide language-independent embeddings.
- Translation APIs: Tools like Google Translate standardize text into a common language for analysis.
Simple implementation: Multi-lingual sentiment analysis implementation using Python.
from transformers import pipeline
Load Multilingual Sentiment Analysis Model
classifier = pipeline(“sentiment-analysis”, model=”nlptown/bert-base-multilingual-uncased-sentiment”)
texts = [ “The product is fantastic!”, # English
“¡El producto es excelente!”, # Spanish
“Le produit est incroyable !” # French
]
Perform Sentiment Analysis
for text in texts:
result = classifier(text)
print(f”Text: {text} => Sentiment: {result[0][‘label’]} with score: {result[0][‘score’]:.2f}”)
Output:
Text: The product is fantastic! => Sentiment: 5 stars with score: 0.98
Text: ¡El producto es excelente! => Sentiment: 5 stars with score: 0.97
Text: Le produit est incroyable ! => Sentiment: 5 stars with score: 0.96
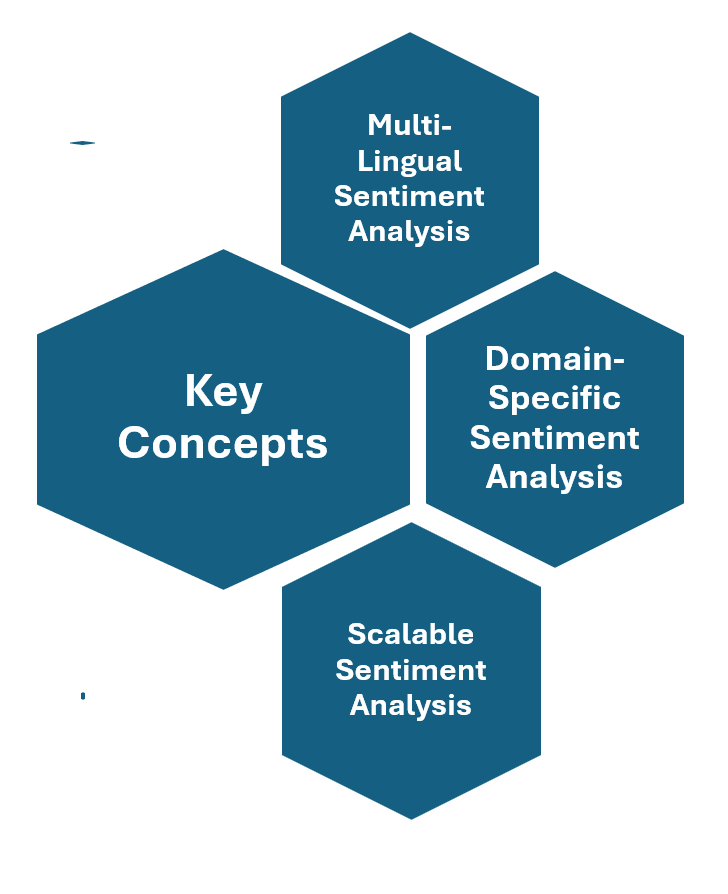
Domain-specific sentiment analysis:
Domain-Specific Sentiment Analysis focuses on interpreting and categorizing sentiments within a particular industry, such as healthcare, finance, retail, manufacturing, education, etc. Unlike general sentiment analysis, it tailors algorithms and models to understand the nuanced language, terminology, and context unique to a specific domain we focus on. Without any questions, this approach provides deeper insights, enabling businesses, strategies and researchers to make informed decisions, optimize services, and address customer or stakeholder needs more effectively and on time. It bridges the gap between generic sentiment interpretation and specialized requirements, ensuring precise and actionable outcomes.
- Use Cases of Domain-Specific Sentiment Analysis
- Healthcare: Analyze patient feedback from reviews, surveys, or social media to identify areas for improvement and enhance patient care. For example, Monitoring sentiments about hospital services or telemedicine experiences.
- Finance: Evaluate customer sentiments regarding banking services, investment products, or market trends to improve offerings and mitigate risks. For example, Identify concerns in customer reviews about a new credit card feature or loan service.
- Retail and E-commerce: Assess product reviews and customer feedback to optimize inventory, improve user experiences, and refine marketing strategies. For example, Detect negative sentiment trends for specific products and adjust inventory or quality accordingly.
- Education: Monitor student or faculty feedback on courses, teaching methods, or facilities to improve educational services. For example, Analyze sentiment in course feedback forms to adapt curriculum or teaching styles.
- Hospitality and Travel: Evaluate guest reviews and travel feedback to enhance services and maintain a competitive edge. For example, Identify issues in hotel reviews to improve customer satisfaction.
- Entertainment: Assess audience reactions to movies, TV shows, or live events across social media and review platforms to refine content strategies. For example, Gauge public sentiment about a newly released film to adjust marketing campaigns.
- Techniques:
- Domain-Specific Training Data: Models trained on specialized corpora, such as financial news or healthcare feedback.
- Custom Lexicons: Creating dictionaries of domain-specific terms and their associated sentiment.
Simple implementation: Domain-specific sentiment analysis implementation using Python.
from transformers import AutoTokenizer, AutoModelForSequenceClassification, pipeline
Load FinBERT Model for Financial Sentiment Analysis
tokenizer = AutoTokenizer.from_pretrained(“yiyanghkust/finbert-tone”)
model = AutoModelForSequenceClassification.from_pretrained(“yiyanghkust/finbert-tone”)
classifier = pipeline(“sentiment-analysis”, model=model, tokenizer=tokenizer)
Financial News Headlines
financial_texts = [
“Stock prices surged after the announcement.”,
“Investors are concerned about potential losses.”
]
Perform Sentiment Analysis
for text in financial_texts:
result = classifier(text)
print(f”Text: {text} => Sentiment: {result[0][‘label’]} with score: {result[0][‘score’]:.2f}”)
Output:
Text: Stock prices surged after the announcement. => Sentiment: Positive with score: 0.94
Text: Investors are concerned about potential losses. => Sentiment: Negative with score: 0.89
Scalable sentiment analysis:
Scalable Sentiment Analysis is the application of advanced natural language processing (NLP) techniques to analyze vast amounts of textual data across multiple platforms in real-time. It enables businesses to process customer feedback, social media posts, reviews, and surveys on a large scale, providing actionable insights into public opinion and trends. With scalability, sentiment analysis can adapt to growing data volumes, diverse languages, and varying domains, ensuring organizations stay responsive and informed. This capability is essential for industries aiming to enhance customer satisfaction, optimize marketing strategies, and maintain a competitive edge in data-driven environments.
- Use Cases of Scalable Sentiment Analysis
- Brand Reputation Management: Monitor sentiment across millions of social media posts and reviews to detect shifts in brand perception in real-time. For example: Identifying and addressing negative feedback during a product recall or PR crisis.
- Customer Experience Optimization: Analyze feedback from customer surveys, support tickets, and live chat sessions to identify trends and improve services. For example, Enhance customer support processes by identifying recurring complaints or areas of dissatisfaction.
- Market Research: Process data from multiple sources like forums, blogs, and news articles to understand consumer sentiment toward a market segment or trend. For example: Gauging sentiment on sustainable products to inform green marketing campaigns.
- Product Development and Innovation: Analyze reviews and user feedback at scale to identify desired features or pain points in existing products. For example: Using customer sentiment to guide the design of a new app feature or hardware update.
- Political and Social Opinion Analysis: Analyze news, social media, and forums to track public sentiment around elections, policies, or social movements. For example: Monitoring public opinion on a new legislative bill or social campaign.
- Global Sentiment Monitoring: Perform sentiment analysis across multiple languages and regions to understand global perspectives on a product or event. For example: Tracking international reactions to a global product launch.
- Techniques:
- Distributed Computing: Tools like Apache Spark for parallel processing of data.
- Real-Time Analytics: Using streaming platforms like Kafka for instant sentiment updates.
Simple implementation: Scalable Sentiment Analysis implementation using Python.
Sample 1: Context: Tracking brand reputation on Twitter.
Input:
• Tweet 1: “I love the new features of this app. Amazing update!”
• Tweet 2: “The update ruined everything. This app is useless now.”
from transformers import pipeline
Load a pre-trained sentiment analysis model
classifier = pipeline(“sentiment-analysis”)
tweets = [
“I love the new features of this app. Amazing update!”,
“The update ruined everything. This app is useless now.”
]
Perform Sentiment Analysis
for tweet in tweets:
result = classifier(tweet)
print(f”Tweet: {tweet} => Sentiment: {result[0][‘label’]} with score: {result[0][‘score’]:.2f}”)
Output:
Tweet: I love the new features of this app. Amazing update! => Sentiment: Positive with score: 0.99
Tweet: The update ruined everything. This app is useless now. => Sentiment: Negative with score: 0.97
Sample 2: Customer Feedback Analysis (E-Commerce)
Context: Analyzing product reviews to identify customer satisfaction.
Input:
• Review 1: “The product quality is excellent, and delivery was fast!”
• Review 2: “Poor quality and delayed delivery. Disappointed.”
Implementation:
Copy code
reviews = [
“The product quality is excellent, and delivery was fast!”,
“Poor quality and delayed delivery. Disappointed.”
]
Sentiment Analysis
for review in reviews:
result = classifier(review)
print(f”Review: {review} => Sentiment: {result[0][‘label’]} with score: {result[0][‘score’]:.2f}”)
Output:
Review: The product quality is excellent, and delivery was fast! => Sentiment: Positive with score: 0.98
Review: Poor quality and delayed delivery. Disappointed. => Sentiment: Negative with score: 0.95
Conclusion
Sentiment analysis at scale is a powerful tool for businesses and researchers, enabling multi-lingual and domain-specific insights across industries. Organizations can decode emotions, understand trends, and make informed decisions by leveraging advanced NLP models and scalable frameworks. Despite challenges, sentiment analysis remains indispensable in today’s data-driven world.